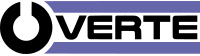 |
Overte C++ Documentation
|
|
12 #ifndef hifi_controllers_AxisValue_h
13 #define hifi_controllers_AxisValue_h
15 #include <QtCore/qglobal.h>
17 namespace controller {
23 quint64 timestamp { 0 };
27 AxisValue(
const float value,
const quint64 timestamp,
bool valid =
true);
29 bool operator ==(
const AxisValue& right)
const;
30 bool operator !=(
const AxisValue& right)
const {
return !(*
this == right); }